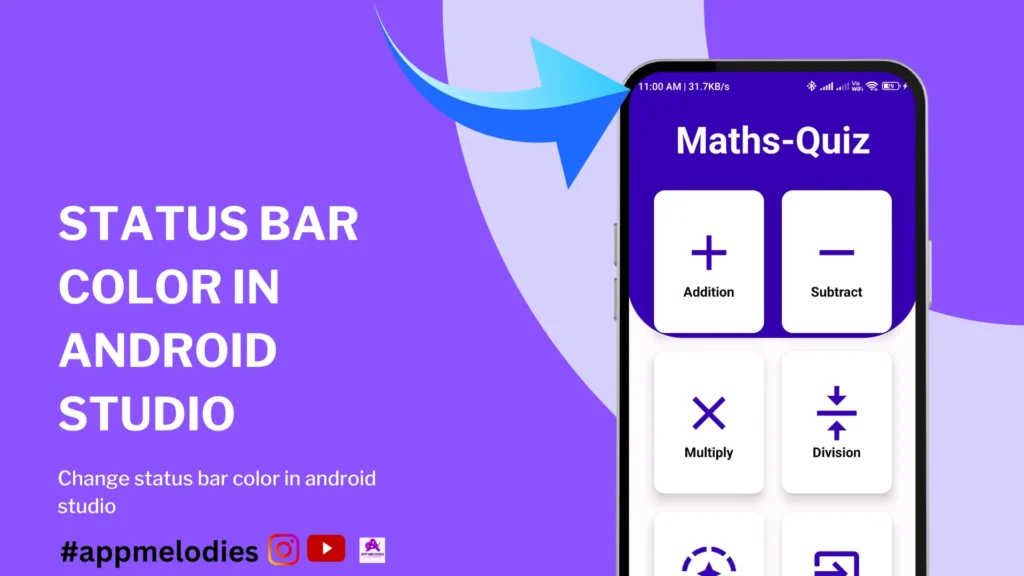
In Android development, creating visually appealing and cohesive user interfaces is essential for a positive user experience. One way to enhance the look and feel of your Android app is by customizing the Android status bar color. In this tutorial, we’ll explore how to programmatically change the status bar color using Kotlin.
Why Customize the Android Status Bar Color?
The status bar is the horizontal bar at the top of an Android device’s screen, displaying important information such as time, battery level, and notifications. By default, Android assigns a system-wide color to the status bar. However, customizing this color can help your app stand out, maintain brand consistency, and provide a more immersive user interface.
Step 1: Project Setup
Assuming you have an existing Android Studio project, open your MainActivity.kt
file.
Step 2: Change the Status Bar Color Programmatically
Inside the onCreate
method, where your main activity is initialized, add the following code snippet:
import android.os.Build
import android.os.Bundle
import android.view.Window
import android.view.WindowManager
import androidx.appcompat.app.AppCompatActivity
class MainActivity : AppCompatActivity() {
override fun onCreate(savedInstanceState: Bundle?) {
super.onCreate(savedInstanceState)
setContentView(R.layout.activity_main)
// Change the status bar color
changeStatusBarColor("#3498db") // Replace with your desired color code
}
private fun changeStatusBarColor(color: String) {
if (Build.VERSION.SDK_INT >= Build.VERSION_CODES.LOLLIPOP) {
val window: Window = window
window.addFlags(WindowManager.LayoutParams.FLAG_DRAWS_SYSTEM_BAR_BACKGROUNDS)
window.statusBarColor = android.graphics.Color.parseColor(color)
}
}
}
Replace "#3498db"
with the hexadecimal color code of your choice.
Step 3: Run Your App
Build and run your app to see the changes in action. The status bar at the top should now reflect the color you specified in the code.
Conclusion
Customizing the Android status bar color in Android apps can be a simple yet impactful way to enhance the overall aesthetic. Whether you’re aligning with your brand’s color scheme or creating a unique visual identity, taking control of the android status bar color adds a personal touch to your app.
In this tutorial, we’ve walked through the process of changing the status bar color programmatically using Kotlin. Experiment with different color codes to find the perfect match for your app’s design. Happy coding!
FAQs
Q1: Can I use this method for older Android versions? A: This method is effective for Android versions Lollipop (API level 21) and above. Ensure your app’s minimum SDK version is set accordingly.
Q2: How can I choose the right color code? A: You can use online color pickers or design tools to find the hexadecimal code that matches your desired color.
Q3: Can I animate the status bar color change? A: Yes, you can use animation techniques to create a smooth transition when changing the status bar color. Explore Android’s animation API for more details.
Q4: Does changing the status bar color impact app performance? A: No, changing the status bar color programmatically has minimal impact on app performance. It’s a lightweight customization.
Q5: Can I set a transparent status bar? A: Yes, you can achieve a transparent status bar by setting the color code to a transparent or semi-transparent value.
Q6: Is it possible to change the status bar color dynamically based on app state? A: Absolutely. You can modify the color dynamically based on user interactions, app events, or specific conditions in your code.
Q7: Are there any guidelines for choosing status bar colors for better visibility? A: Consider using contrasting colors to ensure good visibility. Test your chosen colors to ensure readability and a pleasing aesthetic.
Q8: Can I change the status bar color separately for different screens in my app? A: Yes, you can dynamically change the status bar color based on the specific screen or activity by implementing the color change logic in each activity.
Q9: Does changing the status bar color affect dark mode? A: The status bar color can be adjusted to complement the dark or light mode of your app, ensuring a consistent and visually appealing experience for users.
Q10: Can I revert the status bar color to the system default programmatically? A: Yes, you can revert to the system’s default status bar color by resetting the color to the default value. This is useful for ensuring consistency with the user’s device settings.
You may also like these posts
- 5 Step-by-Step Guide: Create an Exciting Sky-Shooting Game in Android Studio with Kotlin
- How to Develop a Ludo Game in Android Studio Using Kotlin: 7 Steps with Free Source Code Included!
- [ Solved ] Icons not loading in the vector assets section in Android Studio [ 100% Solved ]
- Build an Impressive File Manager App in Android Studio with Kotlin | Free Source Code & 11-Step Guide
- [ Solved ] Unexpected tokens (use ‘;’ to separate expressions on the same line) | Android Studio Error 2 Fixes